2023. 2. 9. 09:12ㆍ개발/Node
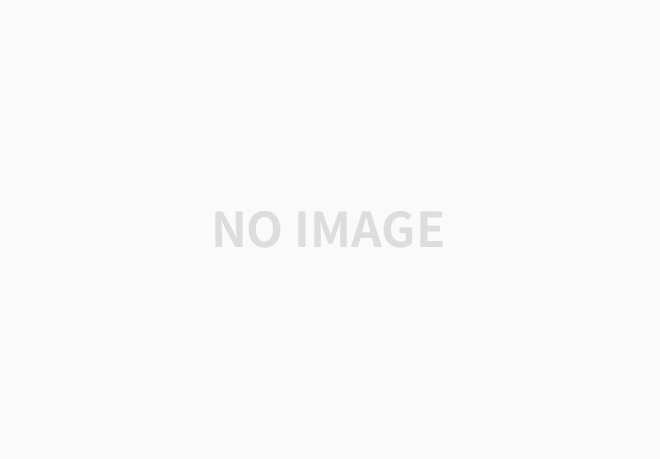
기존에는 app.module.ts에 database 설정을 해놓고 쓰고 있었는데요.
구조 정리를 하면서 env도 사용하고 구조도 나누게 되었습니다.
처음에 사용하던 방식은 공식 문서에 나오는 방법입니다.
Documentation | NestJS - A progressive Node.js framework
Nest is a framework for building efficient, scalable Node.js server-side applications. It uses progressive JavaScript, is built with TypeScript and combines elements of OOP (Object Oriented Progamming), FP (Functional Programming), and FRP (Functional Reac
docs.nestjs.com
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
@Module({
imports: [
TypeOrmModule.forRoot({
type: 'mysql',
host: 'localhost',
port: 3306,
username: 'root',
password: 'root',
database: 'test',
entities: [],
synchronize: true,
}),
],
})
export class AppModule {}
저는 여기서 databse에 대한 설정을 분리하고 .env을 사용하기 위해 수정을 하게 되었습니다.
config 파일 분리
먼저 저는 config폴더를 만들고 database.config.ts를 생성했습니다.
아래 공식문서에서 TypeOrmConfigService class를 만들어서 사용하는 모습을 따라했습니다.
https://docs.nestjs.com/techniques/database#async-configuration
@Injectable()
class TypeOrmConfigService implements TypeOrmOptionsFactory {
createTypeOrmOptions(): TypeOrmModuleOptions {
return {
type: 'mysql',
host: 'localhost',
port: 3306,
username: 'root',
password: 'root',
database: 'test',
entities: [],
synchronize: true,
};
}
}
app.module.ts의 변경점도 같이 나와 있습니다.
TypeOrmModule.forRootAsync({
imports: [ConfigModule],
useExisting: ConfigService,
});
다만 저는 useExisting이 아닌 useClass로 사용을 했습니다.
env 사용을 위한 configService 적용
일단 config를 설치 하지 않았다면 @nesetjs/config를 설치합니다.
$ npm i --save @nestjs/config
app.module.ts에 configservice 설정을 하고,
아까 만든 database.config.ts에 configService를 설정한 뒤 .env 내용을 가져오면 됩니다.
저는 isGlobal 옵션이나 process.env를 사용하지 않으려고 했습니다.
혹시 다른 옵션에 대한 사용이 필요하다면 Configuration에 관한 공식문서를 참고해보면 좋을 것 같습니다.
Documentation | NestJS - A progressive Node.js framework
Nest is a framework for building efficient, scalable Node.js server-side applications. It uses progressive JavaScript, is built with TypeScript and combines elements of OOP (Object Oriented Progamming), FP (Functional Programming), and FRP (Functional Reac
docs.nestjs.com
결론적으로 제가 만든 app.module.ts, database.config.ts, .env 파일을 아래와 같습니다.
@Module({
imports: [
ConfigModule.forRoot(),
TypeOrmModule.forRootAsync({
imports: [ConfigModule],
useClass: TypeOrmConfigService,
}),
],
providers: [AppService],
controllers: [AppController],
})
export class AppModule {
constructor(private dataSource: DataSource) {}
}
@Injectable()
export class TypeOrmConfigService implements TypeOrmOptionsFactory {
constructor(private configService: ConfigService) {}
createTypeOrmOptions(): TypeOrmModuleOptions {
return {
type: 'mysql',
host: this.configService.get<string>('DB_HOST'),
port: +this.configService.get<number>('DB_PORT'),
username: this.configService.get<string>('DB_NAME'),
password: this.configService.get<string>('DB_PASSWORD'),
database: this.configService.get<string>('DB_DATABASE'),
entities: ['dist/**/**/*.entity.{ts,js}'],
synchronize: false,
};
}
}
DB_HOST=
DB_PORT=
DB_NAME=
DB_PASSWORD=
DB_DATABASE=
제가 공식문서와 블로그를 찾아보면서 느낀 점은 '정말 다 다르다' 였습니다.
개발은 어쩔 수 없이 취향이란게 있는 부분이다 보니 본인에게 맞는 부분으로 적용하면 좋을 것 같습니다.
'개발 > Node' 카테고리의 다른 글
NestJS, typeORM relation에서 다른 컬럼 이름을 사용하기 (0) | 2022.10.31 |
---|---|
NestJS, mailgun 이용하기 (0) | 2022.10.12 |
NestJS, DynamoDB에 있는 글들을 hbs에서 보여주기 (0) | 2021.07.27 |
NestJS, 과제 사이트를 만들고 난 느낌 (0) | 2021.07.07 |
NestJS, 파일 읽기 (0) | 2021.06.24 |